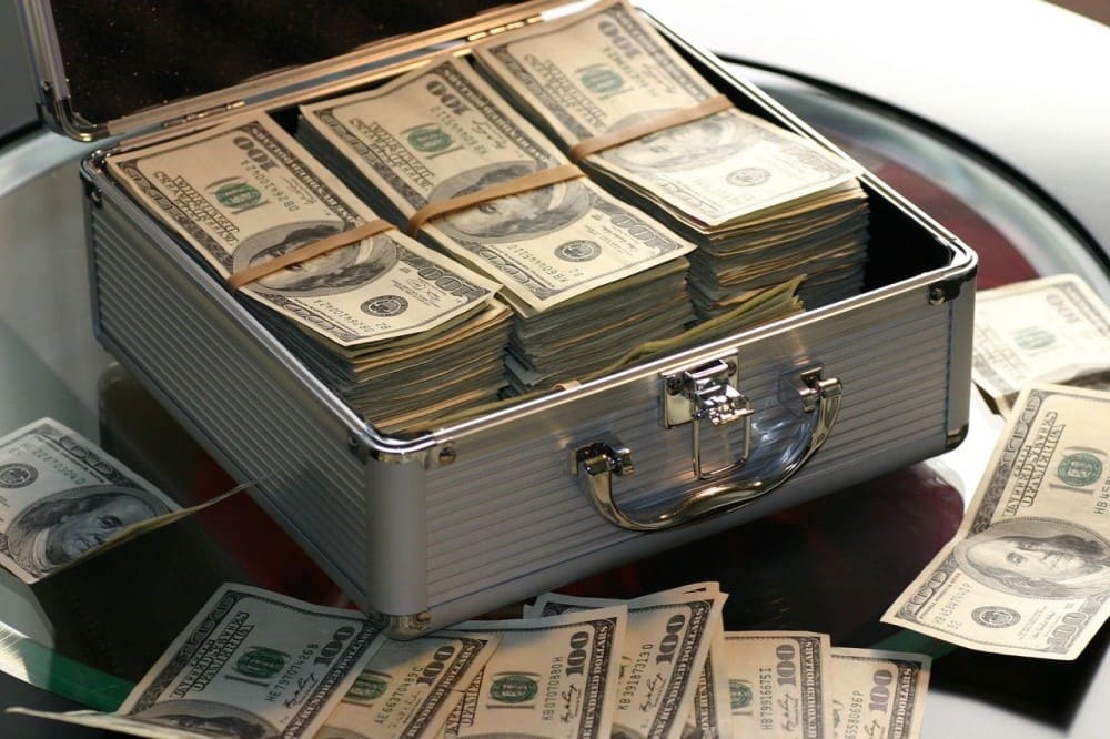
Implementing FX trade decisions with AI
In my previous article, I investigated data on the relationship between FX (currency exchange) rates and breaking news. In this article, I will …
This kind of verification has probably been done by many people over the years, but I’ll try to write about it here. This time, programming is irrelevant. In fact, Python is also irrelevant.
I am a person who melted a lot of money in Forex a little over 10 years ago. It was only a few million yen, but for a young me, it was enough to make me hang myself. Although many months have passed since then, I still feel that it is impossible to win in Forex due to the trauma of that time. In the following article, I am examining the use of AI to trade forex, but I am keeping the investment amount very small.
I don’t ever want to wake up in the morning and find that I’ve lost several months of my salary after gathering all kinds of information and using technical analysis to confidently take a position. The following is something that every trader knows, but I’m going to write about what I’ve noticed.
Let’s consider the case of making a random decision by tossing a coin without thinking about the position. Then, I will investigate how much the expected value will be in a huge number of trials.
First of all, I prepared the following CSV data for the dollar-yen per minute exchange rate. The period is 10 years from 2012/1/1 to 2021/12/31.
USDJPY,20190212,221000,110.46,110.46,110.46,110.46,4
USDJPY,20190212,221100,110.46,110.46,110.46,110.46,4
USDJPY,20190212,221200,110.46,110.46,110.46,110.46,4
USDJPY,20190212,221300,110.46,110.46,110.46,110.46,4
USDJPY,20190212,221400,110.46,110.46,110.46,110.46,4
USDJPY,20190212,221500,110.46,110.46,110.46,110.46,4
...
Then load and use as follows.
def get_csv_data():
with open(f'USDJPY.csv') as f:
reader = csv.reader(f)
rate_arr = []
for row in reader: rate_arr.append(row)
return rate_arr
The next step is to use a random number in python to randomly decide whether to go long, short, or do nothing, with 1 being long and -1 being short.
def get_desition():
rv = random.random()
if rv < (1 / 360): return 1
elif rv >= (1 - (1 / 360)): return -1
return 0
This time, we will make a decision once every minute, so the probability of going long or short the dollar is once every six hours.
And then close the position exactly 6 hours later without any stop loss.
def get_profit(csv, i, desition):
spread = 0
start = float(csv[i][3])
last = i + 360
if last >= (len(csv) - 1):
last = len(csv) - 1
end = float(csv[last][3])
spread = (end - start) * desition
return spread, last
The details of the code are not important, but the point is that the function returns the range of profit and loss for a 6-hour position and the index at the time of closing.
Let’s simulate it with the following code. And calculate the average of the final profit and loss with 1000 trials. Note that we will ignore spread fees and swaps.
initial = 10000000 # Initial investment of 10 million yen
leverage = 3 # Leverage 3 times
unit = 10000 # Trading currency unit: 10000 currency
trials = 1000
if __name__ == '__main__':
csv_data = get_csv_data()
amount_sum = 0
for n in range(trials):
amount = initial
last = 0
for i, c in enumerate(csv_data):
if i < last: continue
desition = get_desition()
if desition == 0: continue
rate = float(csv_data[i][3])
position_unit = math.floor((asset_sum * leverage) / (unit * rate))
if position_unit == 0: break # leaving
spread, last = get_profit(csv_data, i, desition)
amount += position_unit * unit * spread
amount_sum += math.floor(amount)
print(amount_sum / trials)
The result of investing 10 million yen 10 years x 1000 times is as follows.
average balance | expected value | Temporary maximum asset amount | Temporary minimum asset amount | Number of leaving |
---|---|---|---|---|
+98,232 Yen | 100.98% | 73,534,300 Yen | 1,119,500 Yen | 0 |
It’s so obvious that it makes no sense at all, but of course the expected value converges to 100%. If you take into account the actual spread commission, the expected value is a little lower. However, it is much better than my poor investment performance. Moreover, he has increased his assets more than 7 times, albeit temporarily. I don’t know if there is such a thing as a person who can mindlessly plunge 10 million yen into random FX trading.
Leaving aside the smart people who actually trade with 3 times leverage, many junkies who touch FX may trade with 10-20 times leverage, or even more than 100 times leverage at overseas FX companies depending on the person. So let’s try to calculate the case of 10x, 20x and 100x leverage for 10 years x 1000 times under the exact same conditions.
leverage = 20 # 10 or 20 or 100
leverage | average balance | expected value | Temporary maximum asset amount | Number of leaving |
---|---|---|---|---|
10x | -2,666,752 Yen | 73.3% | 714,204,000 Yen | 135 |
20x | -8,745,217 Yen | 12.5% | 3,734,987,699 Yen | 847 |
100x | -9,994,643 Yen | 0.05% | 14,572,122,800 Yen | 1000 |
As mentioned above, if you are trading dollar-yen at 3x leverage, you will have about 100% expected random probability, but as soon as you increase the leverage to 10x, the expected probability becomes 73%. And when you increase the leverage to 100 times or so, you lose all your money, 100% of it. There is no way that ordinary people like you, including me, can make good investment decisions on when and at what rate to go long or short. You may be able to make a slightly better position decision than random (100% expected value), but when you are leveraged more than 10 times, you should think that your expected value will be less than 100%. Moreover, it is very common to make wrong investment decisions when you have emotions.
If there is no unit of trading currency (usually 10,000), the expected value should converge to 100% probabilistically even if the leverage is high. Assuming that you can trade at $0.0001 or 0.0001 yen, you should be able to keep trying without exiting, which means that the expected value should mathematically be 100%. However, the larger the leverage, the larger the volatility, so it will not converge in a small number of trials. To prove this, I checked with 10x leverage and 1 trading unit, and the results were as follows.
leverage = 10
unit = 1
leverage | average balance | expected value | Temporary maximum asset amount | Number of leaving |
---|---|---|---|---|
10x | -316,019 Yen | 96.8% | 3,004,282,686 Yen | 0 |
Even with 10 times leverage, the expected value can be close to 100% without exiting the market. However, if the leverage is 20 times or more, the probability will not converge without a large number of trials, and as mentioned above, the impact of even one trading unit is not negligible (a decimal point in the trading unit is required).
In summary, we believe that most ordinary people will not be able to make a profit in Forex due to the following
By the way, how does the expected value change when you take measures such as stop-loss or stop-loss profit fixing (fixing profit by fishing up the stop-loss line)? The above was settled 6 hours after taking the position without question, but if the rate moves to a loss of 0.25%, the loss is cut, and if there is an unrealized profit of 0.35% or more, the expected value if the rule is to raise the stop line for profit fixing with the rate fluctuations will be as follows.
average balance | expected value | Temporary maximum asset amount | Number of leaving | |
---|---|---|---|---|
-1,136,520 | 88.6% | 79,300,899 Yen | 0 |
Contrary to my expectation, the expected value was much lower than “forced settlement after 6 hours”. I think stop-losses and such are absolutely important, but when you start trading randomly, it may have a negative impact.
People who can trade within 3 times leverage, collect and analyze a lot of information every day, and trade emotionlessly like a machine will be able to make a profit. I can’t do that. If I were to trade with a leverage of 1~2 times, I would have to research a lot of economic information, take a position with an investment amount of about 1 million yen, stare at the rate with anxiety, and if I were lucky enough to get a 1 yen spread between the dollar and yen, I would only make 10,000 yen. I can’t do this. Even if I increase my investment, I’m the type of person who can’t stay in a position when I have “unrealized profit of several million yen,” and I’ll fix my profit.
For an ordinary person like me, it means that forex is entertainment gambling like pachinko or horse racing. It would be better for me to improve the accuracy of system trading, at least as shown in the following article.
In my previous article, I investigated data on the relationship between FX (currency exchange) rates and breaking news. In this article, I will …
I don’t trust almost everything about forex system trading. I don’t know if you can make money with a system that was developed by a huge …