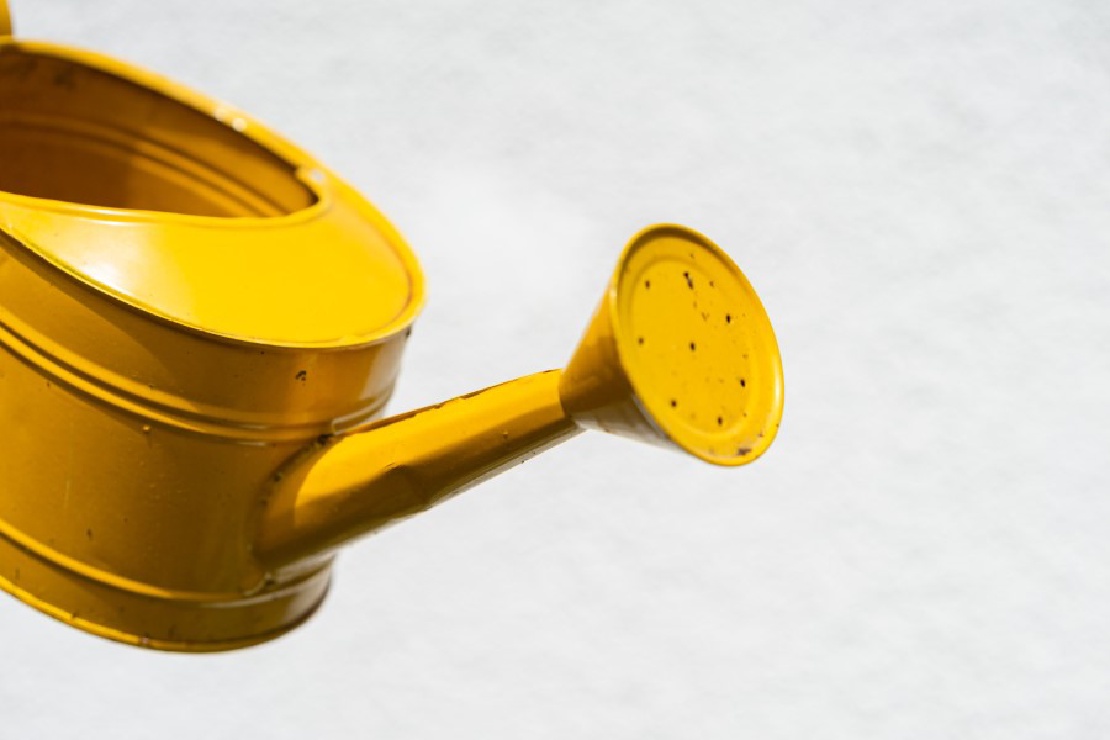
Refactoring helps you grow
Menu In my last article, I introduced that any beginner can implement a “voting system (front-end)”, but of course, if you …
I’ve often been told to keep the readable code in mind. That’s what I’ve often been told. In this site, I have written a lot about the importance of programming without worrying so much about how to write processes. When you are a beginner, it is more important to create something than to know about lambda expressions and interfaces. On the other hand, writing readable code is a different story. How readable the code is is not about how to write the process, but simply how readable it is. In this article, I would like to teach you a small part of this readable code. And this ability will also make a big difference in your programming life.
If you are writing code in a dirty way, as described below, others may not be able to understand it when they see it. To be precise, it’s not that they can’t understand it, it’s that they don’t want to read it because it’s costly to understand. It may also be misleading. This makes it less maintainable.
I’m not going to show my code to anyone! I’m the only one who tweaks the code!" You’re lonely. Do you really think your future self will be able to understand your dirty code? Honestly, I can’t even remember the code I wrote 6 months ago. In other words, it’s important to be aware of readable code for your future self.
It is definitely better to have good English skills when programming. A lot of useful information is written in English. In particular, stackoverflow is a great resource for everyone. Also, when writing code, as I will explain later, the better your English language skills are, the easier it is to write code that is readable for everyone. Well, it is possible to program in Japanese, but considering the editor’s suggestion function, it is better to use English.
If you are not good at English, don’t worry. If you are not good at English, don’t worry, you can just use auto-translation to read English sites. Also, there are only a few English words and phrases that come up in programming, so you will quickly improve your English vocabulary related to programming as you go along. Anyway, you have to make sure that you are not bad at English.
I’ve prepared the following dirty code to exaggerate, but rarely do you encounter people who write code like this in the real world. By the way, this is an example of C# code, but don’t worry, even if you don’t know anything about C#, you can understand what readable is.
public bool CheckUser(int i)
{
// Retrieve user information with matching id from the database
var data = _context.User.where(u => u.Id == i).First();
if (data != null)
{
if (data.Role > 8 || data.HasKey)
{
// Return true because an administrator
return true;
}
}
return false; // Return false because of no permission
}
public async Task RegistUser(User data)
{
// Add a new user
_context.User.Add(data);
// AWAIT the thread and wait for it to be saved.
await _context.SaveChangesAsync();
}
That’s a surprisingly dirty code. If you don’t feel any different when you see this code! Don’t worry! Read this article and you’ll understand it.
By the way, if you don’t use Linq (C# writing style) or async? Please note that I am not saying that these things are dirty. I’m just saying that this code is correct and we should write it in a more readable way.
The word “lame” doesn’t even begin to describe how crappy this is, but the point is that you should name your functions in such a way that you can figure out what they do and what values they contain just by looking at the name.
IsAllowedAdminPage may not be a good function, but I think anyone who reads it will know that it means “do you have permission to access the admin page? CheckUser is a bit too abstract and I don’t know what it’s checking for, and it seems to be conflicting with other functions. By the way, functions and variables that start with “Is~” or “Has~” are widely used because they are named in such a way that you know they are Bool (true/false).
In the same way, the name of a variable should be something that you know what it is the moment you see it. For example, “i” is a variable name that often comes up when doing a “for” statement, but isn’t it the same as the variable i that appears here? If you use “userId”, you can easily find out what the id is. In the same way, the data retrieved from the DB is User data, so the variable name should be user.
This is a very famous story, but it seems that “regist~”, which is intended to mean registration, often appears in the code written by Japanese. It is true that I have seen such naming many times. However, the English word for “register” is “register”. To begin with, I feel uncomfortable using the word “register” in English in programming.
If you want to register a new one, use “Add” or “Create” and everyone will understand. Or, if you don’t want to separate adding and editing, you can use “Save” without any problem.
There are a lot of inexperienced people who write comments like, “You can tell instantly by looking at the code.
「// Retrieve user information with matching id from the database」
>Yeah. I’ll know it when I see it.
「Return true because an administrator」
>Yeah. That’s why you don’t have to explain every single thing because you can see it right below.
If you see comments like this, it makes people think, “This author must be really stupid,” so definitely don’t do that. Also, if you change the process at a later date, do you bother to change the comment as well? What if I forget to change the comment? At any rate, these simple explanatory comments are just harmful. If you are doing something that is not easy to understand at first glance, commenting the reason for it is a meaningful and correct comment, but there are not many cases like that.
You don’t have to write every single process in Japanese in a comment, but you should write Doc comments for functions, methods, and properties. Normally, you would write Doc comments for interfaces, but this time I’m going to comment functions. Doc comments are special comments that define information to create a document, as shown below. This time we will use Doc comments in C#, but of course Doc comments exist in other languages as well, and there are rules on how to write them, so let’s Google them.
/// <summary>
/// Whether or not you have permission to access the administration page.
/// </summary>
/// <param name="userId">user ID</param>
/// <returns></returns>
public bool IsAllowedAdminPage(int userId)
There are two main reasons for each Doc comment.
Swagger to create documentation is very useful, but the functionality displayed in the editor as in 2. is more familiar and convenient. (Displayed as follows)
If you write even a small outline in Doc comments, you can recognize information such as “what kind of method it is” when you try to call it from the outside without having to go to the place where Doc comments are written.
You may or may not like this, but it is best to avoid nesting as much as possible. The following are some examples of nested processes. I think this is the cause of difficulty in reading.
if (is_nest_a)
{
if (is_nest_b)
{
if (is_nest_c)
{
// something
}
}
}
return;
Don’t you think it would be much more readable to rewrite it as follows?
if (!is_nest_a || !is_nest_b || !is_nest_c) return; // Eliminate first.
// something
If the “something” is dozens of lines long, it seems more readable to eliminate the things that don’t need to be processed first, and then let the “something” continue slowly.
There are some people who stupidly add “d” or “i” to their variable names because long names are too annoying to type on the keyboard. Well, what, are you programming in Sakura Editor or something? In today’s editors and IDEs, you can use suggestions, so if you give a name that is easy to understand (and recognize), you can get a list of suggestions after typing a few letters, right? If you are developing in an environment where suggestions are not made, wouldn’t it be more efficient to change that environment first? (I know there are cases where this is not an option, but…)
I know it’s extreme, but it would be nice to have a function named “GetHarryPotterAndThePhillosophersStoneDataWithUserId”. At a glance, it’s easy to see that it’s a function to get the data of Harry Potter and the Philosopher’s Stone, and if you type “GetHarr” or something like that, it will immediately suggest this function, so there’s no need to type the whole thing.
Now, I refactored the code to reflect the above fixes, and the result is as follows. What do you think? Isn’t it easy to understand what the code looks like the moment you see it?
Since there are no unnecessary comments and the naming represents the body, the small number of lines of code will quickly pass through the neurons of your eyes and then quickly pass through the neurons of your brain, and you should be able to recognize what the code represents with fewer calories consumed.
/// <summary>
/// Whether or not you have permission to access the administration page.
/// </summary>
/// <param name="userId">user ID</param>
/// <returns></returns>
public bool IsAllowedAdminPage(int userId)
{
var user = _context.User.where(u => u.Id == userId).First();
if (user == null) return false;
return (user.Role > 8 || user.HasKey);
}
/// <summary>
/// Add new user information
/// </summary>
/// <param name="user">User entities to be saved</param>
/// <returns></returns>
public async Task AddUser(User user)
{
_context.User.Add(user);
await _context.SaveChangesAsync();
}
In summary, readable code is code that is so beautiful that anyone can understand its meaning the moment they see it. As mentioned above, writing readable code means that you can be kind not only to others but also to yourself in the future. In turn, this will greatly improve your programming ability. If you’re not good at English or don’t know words like programming etiquette (like “getter” and “setter”), it’s okay to make mistakes at first. The important thing is to have the will to write readable code.
I know I sound like a big deal, but I still often use “ret” and “tmp” as variable names, with the excuse that “this is a disposable script.
The point is to be aware of the readability as much as possible without being overwhelmed.
Yes. I have been influenced by the following books. I definitely recommend these books as they contain a lot more to learn.
Menu In my last article, I introduced that any beginner can implement a “voting system (front-end)”, but of course, if you …
Menu I will show you how to actually put something together using the programming thought process I introduced in the last article. This …